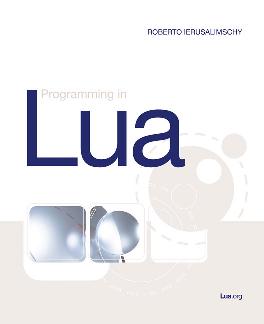
running awesome in archlinux (:
~:$./gkmoney no arguments Current balance: 216 dep 17000 sell a hello world for 17k wit 1500 purchase a old beatle wit 3 beatle crash .. I went back by bus end Tue Mar 2 21:53:18 2010 + 17000 -> 17216 sell a hello world for 17k Tue Mar 2 21:53:34 2010 - 1500 -> 15716 purchase a old beatle Tue Mar 2 21:53:55 2010 - 3 -> 15713 beatle crash .. I went back by bus Current Balance: 15713
#include <stdlib.h> #include <stdio.h> #include <string.h> #include <time.h> int main (int argc, char ** argv) { FILE * bf; FILE * lf; int balance, val, indx, n; char buf[BUFSIZ]; char command[5], * time_str, comment[255]; time_t time_now; if (argc < 2) { printf ("no arguments\n"); bf = fopen ("/home/geckos/.bl.txt", "r+"); lf = fopen ("/home/geckos/.ml.txt", "a+"); } else { bf = fopen (argv[1], "r+"); lf = fopen (argv[2], "a+"); } if (bf == NULL || lf == NULL) { perror ("fopen"); exit (1); } if (fscanf (bf, "%d\n", &balance) == EOF) { perror ("fscanf"); exit (1); } printf ("Current balance: %d\n", balance); while (1) { for (indx = 0; indx < BUFSIZ; indx++) { char ch; n = read (0, &ch, 1); if (n == -1) { perror ("read"); exit (1); } if (ch == '\n') break; buf[indx] = ch; } buf[indx] = '\0'; /* %[^-] = %s + white spaces */ n = sscanf (buf, "%s %d %[^-]", command, &val, comment); time_now = time (NULL); time_str = ctime (&time_now); time_str[strlen (time_str) - 1] = '\0'; /* chop LF */ if (n == 2) memcpy (comment, "...", 4); if (strcmp (command, "dep") == 0) { balance += val; fprintf (lf, "%s\t+%10d -> %10d\t%s\n", time_str, val, balance, comment); } else if (strcmp (command, "wit") == 0) { balance -= val; fprintf (lf, "%s\t-%10d -> %10d\t%s\n", time_str, val, balance, comment); } else if (strcmp (command, "end") == 0) break; } fseek (bf, 0, SEEK_SET); fprintf (bf, "%d", balance); fclose (bf); fseek (lf, 0, SEEK_SET); while (fgets (buf, BUFSIZ, lf)) printf ("%s", buf); fclose (lf); printf ("Current Balance: %d\n", balance); return 0; }